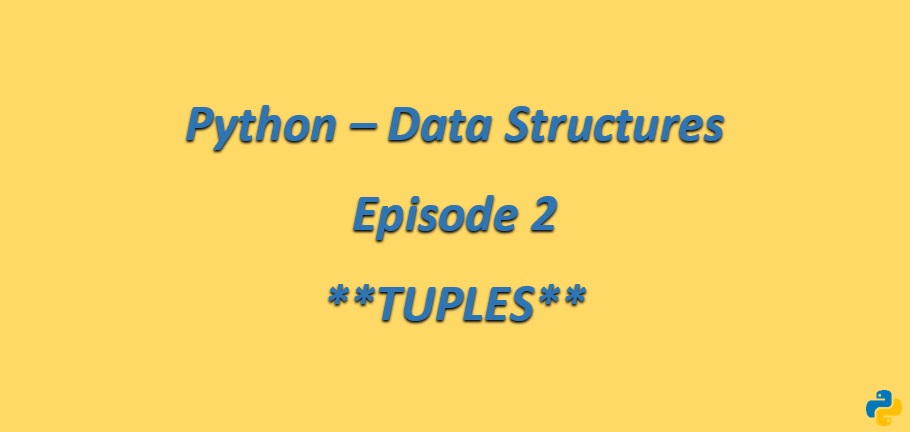
Python - Data Structures - Episode 2 - Tuples
Author : Hamza EL YAAQOUBI
Introduction
In this article, we will explain a new concept introduced in Python. It’s about tuples.
Preview
Before we start talking about tuple, I will introduce briefly two important concepts : sequence types and mutability.
A sequence type is a type of data in Python which is able to store more than one value (or less than one, as sequence may be empty). These values can be sequentially browsed element by element.
The other notion is about mutability is a property of any of Python’s data that describes its readiness to be freely changed during program execution. There are two kinds of Python data: mutable and immutable.
The data type we want to tell you about now is a tuple.
A tuple is an immutable sequence type. It can behave like a list, but it mustn’t be modified in situ.
What is a tuple
Between lists and tuples there is a distinction in the syntax used to create them. Tuples prefer to use parenthesis whereas lists like to see brackets (opened and closest one). Although it’s possible to create a tuple just from a set of values separated by commas.
Look at the example :
tuple1 = (1, 2, 3, 4)
There are one tuple containing four elements.
Let’s print it :
print(tuple1)
Note: each tuple element may be of a different type (floating-point, integer, or any other type).
How to create a tuple
It is possible to create an empty tuple - parentheses are required then :
my_first_empty_tuple = ()
If you want to create a one element tuple, you must end the value with a comma like this :
my_first_one_element_tuple = (1, )
my_first_one_element_tuple = 1.,
Removing the commas won’t spoil the program in any syntactical sense, but you will instead get two single variables, not tuples.
How to use a tuple
You can use the same conventions as lists if you want to get the elements of a tuple in order to read them.
Take a look at the following snippet :
my_first_tuple = (1, 2, 3, 4, 5)
print(my_first_tuple[0])
print(my_first_tuple[-2])
print(my_first_tuple[:])
print(my_first_tuple[2:])
print(my_first_tuple[:-2])
The program should produce the following output :
Because of tuples are immutable you can’t modify a tuple content unlike lists.
So the following instructions will cause runtime error :
my_first_tuple = (1, 2, 3, 4, 5)
my_first_tuple.append(6)
del my_first_tuple[3]
my_first_tuple[1] = 7
This program should produce the following output :
AttributeError: 'tuple' object has no attribute 'append'
Also, with tuple, you can use :
- The len() function that accepts tuples, and returns the number of elements contained inside
- The + operator to join tuples together
- The * operator to multiply tuples, just like lists
- The in and not in operators to work in the same way as in lists
Conclusion
As you can see, we have a new data sequence type that we can use in our programs.
So you can start to play with it because in the next article I will introduce other sequence type, the dictionaries.
Stay tuned and enjoy Python.
To subscribe my newsletter, please fill out the form available on my website.
See you soon.