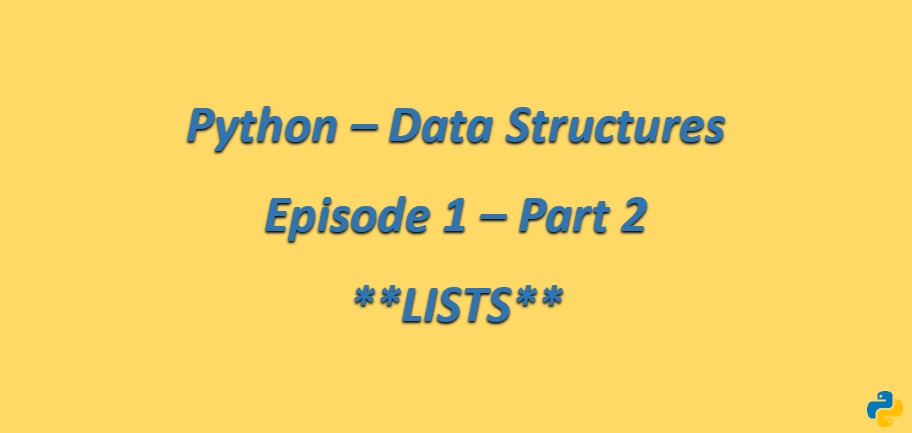
Python - Data Structures - Episode 1 - Lists - Part 2
Author : Hamza EL YAAQOUBI
Introduction
This article is a continuation of the other article Python - Data Structures - Episode 1 - Lists - Part 1.
So I will talk about other operations such as access, search, delete, max, min and len methods. I will also explain the time complexity of each operation.
- Access operation
- Syntax
To access an element, we have to get its number in the list (remember what we saw in the previous article). That number is putted inside the brackets which selects the element of the list, and it is called an index, while the operation of selecting an element from the list is known as indexing.
The syntax is to put the index between the open square bracket with a closed square bracket.
This operation return the value in the list.
- Complexity Time
Such as the index of the element is known, the time complexity is constant
Using the Big O Notation, the time complexity is O(1).
- Example
list = [1, 2, 3]
print(list[0])
print(list[1])
print(list[2])
This snippet outputs :
NB : If the index does not exist in the list, Python raise an exception.
list = [1, 2, 3]
print(list[3])
- Search operation
- Syntax
To search an item in the list, we can use the keyword in to accomplish our search.
This operation return True if the value exists in the list.
element in list
- Complexity Time
This operation can take in the worst case N iteration such as N is the size of the list.
Using the Big O Notation, the time complexity is O(N).
- Example
list = [1, 2, 3]
print(1 in list)
print(2 in list)
print(3 in list)
print(4 in list)
This snippet outputs :
- Delete operation
- Syntax
To remove an item from the list, you can use two way :
del : we use the del statement if we know exactly which element you are deleting.
remove : we use the remove method if we don’t know exactly which elements to delete.
The remove method does not return any value but removes the given object from the list.
del list[index]
list.remove('item_to_be_deleted')
- Complexity Time In the worst case, remove operation can take N iterations.
Using the Big O Notation, the time complexity is O(N).
- Example
list = [1, 2, 3]
del list[0]
print('The list contains the following elements : ', list)
list.remove(2)
print('The list contains the following elements : ', list)
This snippet outputs :
Conclusion
In this article, we discussed how to access element in the list and how to search an item in the list and finally how to delete an element from the list.
Here’s key takeaways for the time complexity :
Thank you for your attention.
Stay tuned !